in this Blog we see how to Create an Object in TypeScript & Assign Properties to the Objects.
Object in TypeScript:
An object is an instance which contains set of key value pairs. The values can be scalar values or functions or even array of other objects.
Syntax:
var object_name = {
key1: “value1”, //scalar value
key2: “value”,
key3: function() {
//functions
},
key4:[“content1”, “content2”] //collection
};
In TypeScript Objects are created in multiple ways .
We can build objects directly without constructing classes by using Object Literals and function Object() { [native code] } methods because JavaScript works fundamentally on template-based code snippets.
Object Literals in Typescript :
Object Literals are defined as a set of name-value pairs stored in comma-separated lists.
Syntax:
var Name_of_object = {
object_property : value,
object_property : value
}
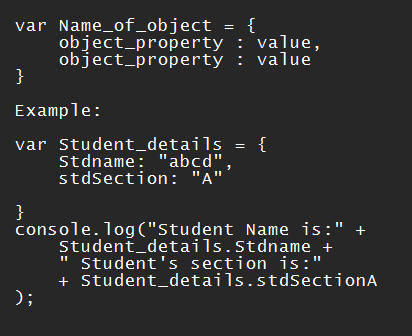
Outptut :
Constructor Method in TypeScript:
A Constructor method is intended primarily for initialising an Object created with a class.
Note: Only one special method can have the “function Object() { [native code] }” status in a defined class, and if more than one function Object() { [native code] } method is added in a class, a SyntaxError will be thrown.
Syntax:
function Name_Of_Constructor( property1, property2, …) {}
Inside this Constructor method, we can initiate parameter values to properties of objects using the “this” keyword.
function Name_Of_Constructor( property1, property2, …) {
this.property1 = parameter_value;
this.property2 = parameter_value;
}
or
We can declare both properties of object and parameter with the same name.
function Name_Of_Constructor( property1, property2, …) {
this.property1 = property1;
this.property2 = property2;
}
“this” Keyword references object property with the required parameters, to simply say “this” keyword represents the object to which we are initiating parameters with help of the constructor method.
Output
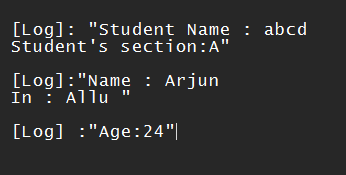
Executing Objects as Parameters to Functions in TypeScript :
Let’s evaluate the method for giving an object to a function as a parameter value. With Typescript, objects can typically be supplied as arguments, however we need add the characteristics that an object must have to that function.
Syntax :
var Name_Of_Object {
property = property.value ;
}
function function_name(
obj : { property_name : property_type }
) : return_type {
obj_param.property
}
Example:
var Student = {
firstname: ” ajay “,
lastname: ” kumar “,
}
function display( obj: {
firstname:String,lastname:String
}) : void {
console.log(“Name is”+obj.firstname+” “+
“lastname is”+” “+obj.lastname);
}
display(Student);
Output :
